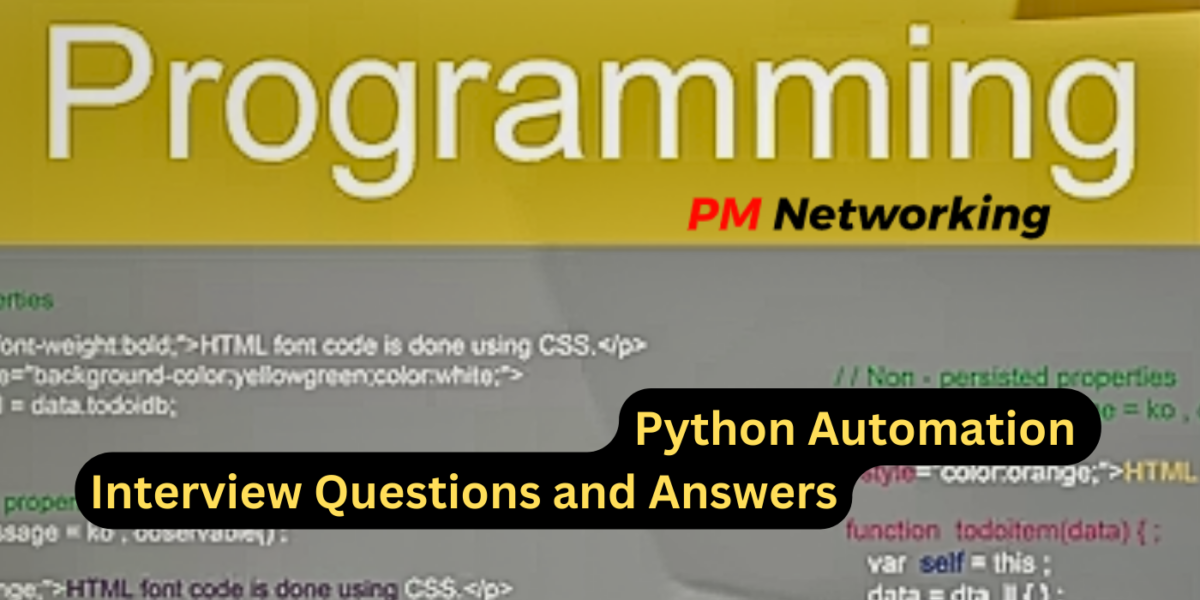
One of the most important aspects of software engineering is software testing. An application that has not been thoroughly tested is likely to contain many bugs and errors. A QA automation engineer ensures that each system works as expected by conducting quality assurance with automation tools and defining standards, processes, and methods. Below is a list of some Python automation interview questions with sample answers that will surely prove helpful during your interview.
Python Automation Testing Interview Questions
First, you will be asked to indicate how experienced you are with software automation testing in general. After that, the technical interviewer will check your practical knowledge of Software Quality Assurance fundamentals and Python automation testing tools.
Get Out the Best Course
Complete Python Automation Online Course for Network Engineers
Cisco CCNA 200-301 Course Online
CCNP (Encor and Enarsi) Online Training Course
CCNP Service Provider | Training and Certifications
1. What is the difference between a test plan and a test strategy?
A test plan is a document that defines the approach, resources, scope, deliverables, objectives, estimates, schedule, etc. of testing activities. A testing strategy is a set of principles or guidelines that explain test design and determine how testing should be conducted.
2. Mention at least 3-4 advantages of using Python compared to other scripting languages like JavaScript.
Some of the benefits of using Python are listed below:
- Application development is fast and easy.
- Comprehensive support of modules for any type of application development including data analytics/machine learning/math-intensive applications.
- The community is always active in resolving user queries.
3. What is flaky testing and how do you work with it?
A test is considered “flaky” if it cannot produce the same result every time it is run without making any changes to the code under test or the test configuration. For example, suppose Test 1 depends on some global configuration. Now we write Test 2 which changes this global configuration, and Test 1 fails. In this scenario, Test 1 is an example of a tiered test.
4. Does Python allow you to program in a structured style?
Yes, Python allows to code in a structured and object-oriented style. It provides excellent flexibility to design and implement the application code based on the requirements of the application.
5. When do you use fixtures?
We use fixtures in testing to ensure that there is a fixed configuration (or environment) for tests so that they can run multiple times. A good example of test consistency is initializing the database with specific credentials for testing.
6. What is PIP software in the Python world?
PIP stands for Python Installer Package, which provides an intuitive interface for installing various Python modules. It is a command-line tool that searches for packages on the Internet and installs them without any user interaction.
7. What is parameterizing?
Parameterizing is running the same test (same test function) multiple times with multiple parameters (inputs). For example, you want to test your function ‘Multi’ which takes 2 numbers and multiplies them. Using test standardization, we have values such as (1, 4), (-1, 0), (‘string’, 3), (True, 12), (none, -1), ({}, (1) )) in our testing to make sure that our ‘multi’ can handle multiple edge cases.
8. How do you use Arrays in Python?
Python does not have in-built data structures like arrays, and it does not support arrays. If you want, you can use a list that can store an unlimited number of elements.
9. What is parallel testing?
Parallel testing, or parallelism, is running a set of tests at the same time. These sets of tests may have similar or different configurations. The main objective of parallel testing is to reduce execution time.
10. Which databases are supported by Python?
MySQL (structured) and MongoDB (unstructured) are the major databases natively supported by Python. Import models using functions to interact with the database.
11. What is the purpose of __init__() function in Python?
It acts as a constructor that is executed when an object of the class is instantiated and allows the class to enumerate its attributes. This is equivalent to the constructor concept in C++.
12. How is a lambda function different from a normal function in Python?
Lambda is similar to the inline function in C programming. It returns a function object. It has only one expression and can accept any number of arguments.
In the case of normal functions, you can define a function name, pass parameters, and essentially have a return statement. But lambda functions are usually used for simple operations without the use of function names. It can also be used in place of variables.
13. Why do we need test documentation?
A set of artifacts prepared before testing is called test documentation. It includes test coverage, terminology, execution process, and all the testing activities that need to happen. Test documentation is important because it shows us which part of our project is important and how to test it. It also helps teams improve, validate, plan, review, and understand tests (and testing processes). This leads to a clear understanding of the goals of the project.
14. What are the differences between CI, CD, and CT?
These are the main differences between CI, CD, and CT:-
CI (Continuous Integration): The process of continuously integrating code into our codebase.
CD (Continuous Delivery or Deploy): Automated pipeline of entire software releases. For example, when someone wants to deploy to a dev environment, this pipeline ensures that tests are passed, the code is reviewed and approved for deployment, and the only remaining step is deployment.
CT (Continuous Testing): This allows engineers to perform deployment and post-deployment testing in certain (local) environments. We can also say that CT runs tests after every commit.
15. What are QGs?
GQ (Quality Gates) can be defined as a milestone for a project that includes several important criteria. If these criteria are not met, the project cannot continue to the next stage.
16. What is the difference between TCP and UDP?
TCP (Transmission Control Protocol) is a connection-oriented protocol that establishes a connection to transfer data and must close it after the transmission is complete.
UDP (User Datagram Protocol) is a connectionless protocol, meaning it does not need to establish, maintain, or terminate a connection, and is therefore much faster than TCP.
17. What is the starting point of Python code execution?
Since Python is an interpreter, it starts reading the codes from the source file and then executes them.
However, if you want to start from the main function, you must have the following special variables set in your source file:
1 if__name__== “__main__
2 main()
18. Name some important modules available in Python.
Networking, Mathematics, Cryptographic Services, Internet Data Handling, and Multi-Threading modules are the major modules. Apart from these, many other modules are available in the Python developer community.
19. Which modules of Python can be used to measure the performance of your application code?
The “time module” in Python is used to calculate time at various stages of the application and the logging module is used to log data to the file system in any preferred format.
20. What is the OSI model and its layers?
The Open Systems Interconnection (OSI) model consists of seven layers that computers use to communicate over networks:
- Physical layer: It is responsible for the actual physical transmission of information flow in the form of bits between devices.
- The data link layer is responsible for transmitting messages from one network node to another network node.
- The network layer is responsible for data transmission from the current network host to another host (in a different network). Furthermore, this layer handles the routing of packets.
- The transport layer receives the data transferred at the session layer, breaks it into “segments” and transmits it to the network layer.
- The session layer establishes communication channels between devices. It also takes care of authentication and security.
- The presentation layer prepares (manipulates) data for the application layer.
- The application layer is used as end-user software.
Browse our website and apply for the exciting jobs that interest you. We hope you will join us from wherever you are. We hope you have gained enough technical knowledge for your interview with these Python QA Automation Interview Questions.
Conclusion
We hope this Python interview questions article helped you understand the nature of popular interview questions related to Python. Please note that in addition to theoretical knowledge, you must have very strong logical and analytical skills to write some Python programs during the interview session. Python automation interview questions are an important tool to assess a candidate’s ability to automate tasks and solve problems with Python. You should use them in conjunction with a wide range of assessment methods to comprehensively evaluate a candidate’s skills, personality, and cultural fit. Best of luck in advance!
0 Comments