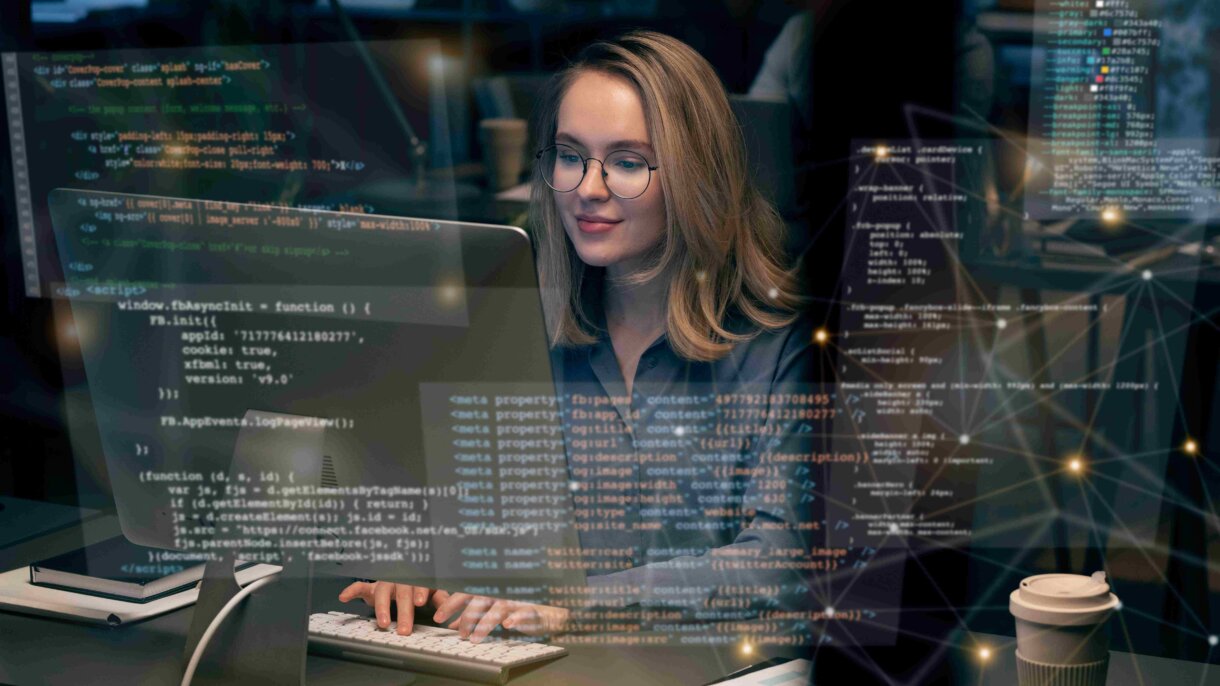
Starting with Python? Welcome to the blog! If you are a beginner who wants to learn the Python basics from scratch or for those who, perhaps, wish to revisit the basics, here are the foundational concepts to get you started with the Python programming language: This is a good Python tutorial for beginners! Do not forget to take some useful Python notes to deepen your knowledge. Let’s start!
Variables
Think of a variable as a container that holds data. In Python, we use the = operator to assign a value to a variable. The value can be anything—like a number, a word, or even a list of things. Once you assign a value to a variable, you can use it throughout your program.
Example:
a = 10
b = “Welcome”
c = 3.1415
Here, a holds the value 10, b holds the string “Welcome,” and c holds the floating-point value 3.1415. Pretty simple, right?
Naming Variables
When it comes to naming your variables, remember this:
- Start with a letter or an underscore.
- After that, you can use letters, numbers, and underscores.
- Python is case-sensitive, so a and A are two different variables.
You can also change the value of a variable at any time. For example:
a = 10
a = 20
Data Types
Data types are just the type of information a variable holds. Python supports a range of types to store different kinds of data.
- Numbers: Integers (1, 2, 3), or floats (3.14, 1.23)
- Strings: A collection of characters, like “python” or “coding”
- Lists: Ordered and changeable collections of items, written in square brackets [5, 10, 15]
- Tuples: Similar to lists, but immutable (unchangeable), written in parentheses (10, 20, 30)
- Dictionaries: Key-value pairs, written in curly braces {name: “John”, age: 30}
- Booleans: True or False
- None: Represents a null value or the absence of a value
Want to know what type of data a variable holds? Use the type() function.
Example:
a = 10
print(type(a)) # Output: <class ‘int’>
b = “Python”
print(type(b)) # Output: <class ‘str’>
Operators
Operators are special symbols that carry out specified actions on one or more operands. In Python, we have various types of operators:
- Arithmetic Operators: Add (+), subtract (–), multiply (*), divide (/), and more.
- Comparison Operators: Compare values—==, >, <, >=, etc.
- Logical Operators: Combine conditions—and, or, not.
- Assignment Operators: Assign new values to variables—=, +=, -=, *=, etc.
- Membership Operators: Check if something is in a sequence—in, not in.
- Identity Operators: Compare objects—is, is not.
Example:
a = 10
b = 20
sum_result = a + b # Adds a and b
print(sum_result) # Output: 30
is_less_than = a < b # Checks if a is less than b
print(is_less_than) # Output: True
Conditional Statements
With conditional statements like if, elif, and else, you can make your code decide what to do based on certain conditions.
Example:
a = 5
if a > 0:
print(“Positive number”)
else:
print(“Not a positive number”)
You can even chain conditions with elif for multiple checks.
a = -5
if a > 0:
print(“Positive number”)
elif a == 0:
print(“Zero”)
else:
print(“Negative number”)
Loops
Loops let you execute a block of code repeatedly. Python has two main types: for loops and while loops.
- For loops iterate over a sequence (like a list, tuple, or string).
- While loops run as long as a condition is True.
Example: For Loop
values = [2, 4, 6, 8]
for val in values:
print(val)
Example: While Loop
x = 0
while x < 3:
print(x)
x += 1
Be cautious with while loops! If the condition never becomes False, you’ll end up in an infinite loop. In order to prevent this, ensure that the condition eventually becomes false.
Functions
Functions allow you to package code into reusable chunks. This makes your code more organized and easier to maintain.
Example:
def greet(name):
print(“Hello, ” + name)
greet(“Alice”) # Output: Hello, Alice
You can also make functions return values with the return keyword.
def add(x, y):
return x + y
result = add(5, 8)
print(result) # Output: 13
And if you want, you can set default values for function parameters.
def greet(name, greeting=”Hi”):
print(greeting + “, ” + name)
greet(“Alice”) # Output: Hi, Alice
Libraries
You can use libraries—pre-written collections of code—to extend your program’s functionality. Some popular libraries include:
- NumPy: For numerical operations
- Pandas: For data manipulation
- Matplotlib: For data visualization
- Scikit-learn: For machine learning
- TensorFlow: For deep learning
- OpenCV: For computer vision
You can install and use these libraries with pip, Python’s package installer.
pip install numpy
Then, import them into your program like so:
import numpy as np
Classes and Objects
Python is object-oriented, which means you can create classes—blueprints for objects. Classes let you model real-world concepts and organize your code better.
A class has attributes (data) and methods (functions). When you create an instance of the class, that’s an object.
Example:
class Car:
def __init__(self, model, year):
self.model = model
self.year = year
def honk(self):
print(“Beep! Beep!”)
car1 = Car(“Toyota”, 2020)
car1.honk() # Output: Beep! Beep!
Conclusion
You have only covered the introduction and basic concepts of Python, and trust me, there is much more out there. It is relatively easier than other programming languages, which makes it a great tool for things like building websites, working with data, or even exploring AI. So, keep learning the Python basics, and remember—practice makes perfect!
This is only the starting point of your journey with the versatile language of Python. Happy coding!
Frequently Asked Questions (FAQs)
- Is the Python tutorial helpful?
Yes, a Python tutorial is very helpful as it provides structured guidance, helping you understand core concepts, practice coding, and build a strong foundation in Python.
- Is it necessary to make Python notes?
Yes, making Python notes is important as it helps reinforce your learning, organize key concepts, and make it easier to review and remember important information.
- What are the Python basics I should learn first?
It’s important to start with Python basics like variables, data types, loops, and functions. Once you’ve grasped these fundamentals, you’ll be ready to dive deeper into more complex topics. - How can I use Python tutorial points to enhance my learning?
Python tutorial point is a fantastic resource for finding tutorials, examples, and explanations on specific Python topics. It’s perfect for expanding your understanding and helping you solve problems efficiently. - Can I learn Python at my own pace?
Yes! The Python tutorial is designed to help you learn at your own pace. Whether you want to go slow or dive in quickly, the resources available, like Python notes and Python tutorial point, will support your learning journey!
0 Comments